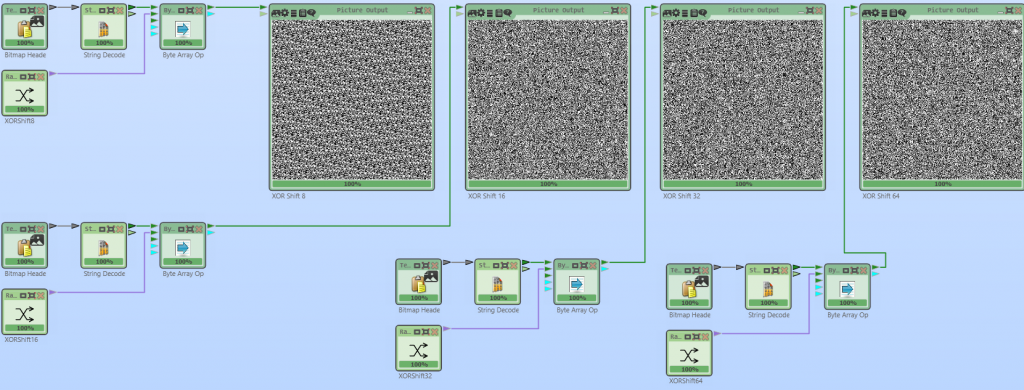
I spend some time on the Random Number Generator component of CrypTool 2. The component allows the generation of random numbers. The output format can be set to byte array, (big) integer, integer array, or just a random bool 🙂
One of my students implemented the component a few years ago. I realized, that the component did not output the random numbers directly. Instead, it created “new” random numbers by a creating single bits per random number. The bits then were used to create the output data. Since people should get what they selected I changed the code. Now, it directly outputs the original random numbers. Also, the generation was not very fast. It “wasted” a lot of bits. You needed to create 8 random numbers for a single 8 bit random number.
Old and new random number generators
Speaking of generators: the component offers different pseudo random number generators. For example linear congruential generator, inverse congruential generator, and x^2 mod N. It offers also the standard .net Random.random as well as the cryptographic random number generator RNGCryptoServiceProvider. For the generation of cryptographic keys and IVs this should always be used.
Additionally, I added the “XORShift” random number generator with 8 bit, 16 bit, 32 bit, and 64 bit. The XORShift takes the previous number and XORes it three times with a shifted value of itself. For example, the 8 bit XORShift can be implemented with five shifts to the left, three shifts to the right, and seven shifts to the left:
_state = (byte)(_state ^ (_state << 5));
_state = (byte)(_state ^ (_state >> 3));
_state = (byte)(_state ^ (_state << 7));
In a similiar fashion, I implemented 16 bit, 32 bit, and 64 bit. Wikipedia contains many other versions of XORShift. These I will probably implement in the near future. For fun, I created a comparision using images of all XORShift implementations we have in CT2. The figure at the beginning of thist blog post shows this comparision. As you can see, XORShift 16 already looks “very” random. That is because of its period of 2^16, meaning, after 2^16 iterations the numbers repeat.
Test it and read more about XORShift
You can test the new XORShift in the new nightly build of CT2. Also, if you are interested in more details about XORShift, I recommend reading the nice Wikipedia article here: https://en.wikipedia.org/wiki/Xorshift. XORShift is also really nice for creating random numbers on old 8 bit machines like the C64. Its easy to implement, fast, and “random enough” for games :-).
Nils