Can you build a cipher with the structure of the Advanced Encryption Standard (AES), our current standard modern symmetric cipher, but only use classical ciphers? I asked myself this question when I implemented AES in C# as a preparation for my upcoming AES videos on my YouTube channel in 2021.
AES’ structure (10 rounds for AES-128) consists of 4 different building blocks:
1) AddRoundKey,
2) SubBytes,
3) ShiftRows, and
4) MixColumns:
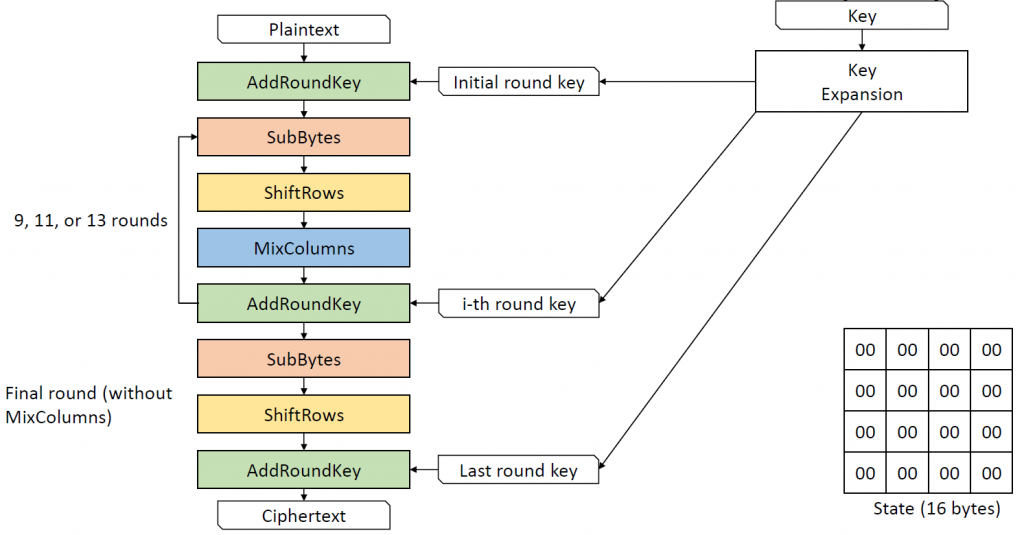
The AddRoundKey building block adds a round key to the state array of 16 bytes (or plain and/or ciphertext) using XOR. The SubBytes building block substitutes each byte using AES’ S-Box, the ShiftRows building block performs a shift of the rows of the state array, and the MixColumns building block mixes the columns of the state array by multiplying each “vector” with an invertible matrix in the finite field GF(2^8).
When I implemented each of these four steps, I was reminded of some classical ciphers: AddRoundKey reminded me of an additive cipher, SubBytes reminded me of a simple substitution cipher, MixColumns reminded me of a transposition cipher, and the matrix multiplication finally reminded me of a Hill cipher.
Thus, I changed the inputs (plaintext and key) and the output (ciphertext) of the AES to simple text (just letters from A to Z), exchanged AddRoundKey with an additive cipher (using MOD 26), exchanged SubBytes by SubBigrams (a bigram substitution cipher), I kept ShiftRows as it was, and exchanged MixColumns with a 4×4 Hillcipher (also using MOD 26). The “TextAES” was born :-).
To also allow decryption, I computed the inverse S-Box (an inverse lookup table for the bigram substitution cipher) and an inverse matrix for the Hill cipher.
I kept the key expansion more or less as it was, but with text, and also used the bigram substitution and replaced its round constants by “AAAA”,”BAAA”,”CAAA”, etc.
Finally, I was convinced that you can create an AES-like cipher using only classical ciphers :-).
If you are interested in details of this self-made crazy cipher, have a look at the video I made about it:
If you are interested in details of the real AES, you may also have a look at my other two videos about AES and AES key schedule:
Also, if you want to play with my source code in C# of AES and TextAES, you can find it freely available on GitHub: https://github.com/n1k0m0/AES-and-Text-Based-AES
Finally, here is the original publication of AES:
Daemen, Joan, and Vincent Rijmen. The design of Rijndael. Vol. 2. New York: Springer-verlag, 2002.
Nils